1. Use Asynchronous Functions
Asynchronous functions are the heart of JavaScript. They perform non-blocking I/O operations, allowing the CPU to handle multiple requests simultaneously.
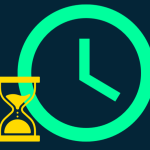
2. Optimize Database Queries
Efficient database queries can significantly reduce response time. Use logging to find which query takes the most time and optimize it.
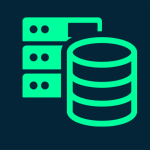
3. Avoid Sessions and Cookies in APIs
Stateless APIs are common and provide JWT, OAuth, and other authentication mechanisms. These authentication tokens are kept on the client side, reducing the load on server.
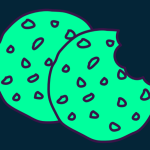
4. Use Caching
Caching can handle frequent common requests, removing the need to make additional queries.
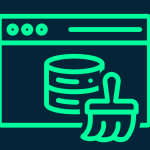
5. Efficient Code and Architecture Design
Breaking your application into smaller, self-contained modules can reduce complexity and make it easier to manage and scale.
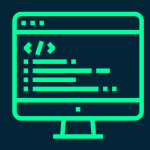
6. Use the Latest Version of Node.js
Newer versions of Node.js generally have performance improvements and bug fixes.
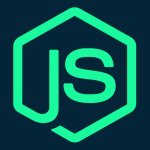
7. Use a Profiler
A profiler can help you identify areas of your code that are causing performance bottlenecks, such as slow function calls or memory leaks.
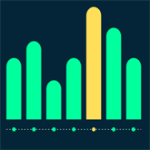
8. Use Throttling
Throttling can prevent your API from being overwhelmed by too many requests at once.
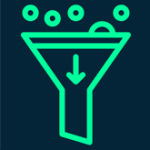
9. Fail Fast with Circuit Breaker
This technique can prevent a function that is likely to fail from being executed, which can improve the overall performance of your API.
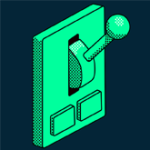
10. Use HTTP/2 Instead of HTTP
HTTP/2 can provide performance benefits over HTTP, such as header compression and multiplexing.
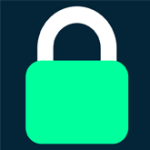
11. Optimize APIs with PM2 Clustering
PM2 is a production process manager for Node.js applications with a built-in load balancer. It allows you to keep applications alive forever and reload them without downtime.
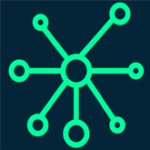
12. Reduce TTFB (Time to First Byte)
This is the time it takes for the client to receive the first byte of data from the server. Reducing TTFB can improve the perceived performance of your API.
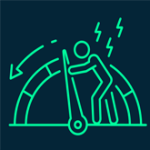
13. Run Tasks in Parallel
This can help to improve the performance of I/O operations.
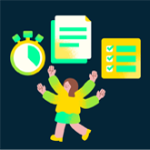
14. Use Error Scripts
with Logging
This can help you quickly identify and fix issues.
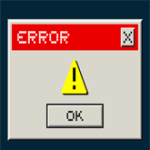